im系统客户端设计
## 客户端用户名片
用于扫码加好友
规则:
业务类型#数据
聊天系统用户名片对应如下
im-namecard#897954546541454&0
im-namecard#897954546541454&1
im-namecard#897954546541454&2
**im-namecard**代表是聊天系统用户名片模块功能, **\#** 是分割符号,**897954546541454&0**中**897954546541454**代表用户id,**0**代表用户类型。用户类型分别有
==0客户 1 门店 2 技师==
## 客户端用户数据存储
客户端用数据存储分为三个本地数据表,分别为本地消息表,本地通讯录表,本地会话表
### 本地消息表
本地消息表是手机端用来本地存储用户聊天记录的表结构,其定义如下:
```javascript
{
id // 本地消息自增长id
msgId //消息唯一id,由服务端生成
channelId // 会话id
channelType // 会话类型 1-单聊 2-群聊
content // 消息内容
msgType // 消息类型 1-文本; 2-图片; 3-语音; 4-视频; 5-文件; 6-富文本; 7-事件
fromUid // 发送人id
fromName // 发送人名称
fromAvatar // 发送人头像
toUid // 接收人id
toName // 接收人名称
toAvatar // 接收人头像
status // 本地消息状态 规定 1-成功 2-失败
unread // 本地消息未读标识 0-已读 1-未读
createAt // 消息创建时间
}
```
### 本地通讯录表
本地通讯录表是手机端用来本地存储用户联系人列表的表结构,其定义如下:
```javascript
{
contactUid // 通讯录好友id
contactAccountId // 通讯录好友账户id
contactName // 通讯录好友名称
contactAvatar // 通讯录好友头像
contactType // 通讯录好友类型 0客户 1 门店 2 技师
contactChannelId // 发送消息时使用的唯一会话id
phoneNumber // 通讯录手机号码
status // 本地通讯录状态 规定 1-对方发起申请 2-本人拒绝申请 3-本人发起申请 4-对方拒绝申请 5-同意(意味着双方都已同意成为好友) 6-本人主动删除 7-被对方删除 8-临时会话生成
reason // 好友申请时提交的申请理由
createAt // 创建时间
}
```
### 本地会话表
本地会话表是记录手机端用来本地存储与联系人或群产生消息交互记录的表结构,其定义如下:
```javascript
id // 会话id
title // 会话名
img // 会话图片
type // 会话类型 1-单聊 2-群聊
lastMsgTime // 最后一次消息的时间
lastMsgContent // 最后一次消息的内容
seq // 排序
unreadCount // 本地会话消息未读条数
status// 本地会话状态 规定 1-正常 2-隐藏
createAt // 创建时间
```
UML
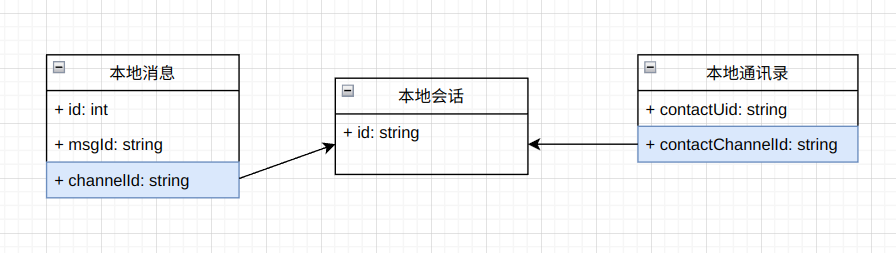
## ChatService类API使用说明
### 1.获取ChatService实例
```javascript
// 传递一个包含三个参数的对象参数
// userId代表当前app登录的用户id,如果是客户端取userid,门店端取storeid
// accountId代表当前app登录的用户账户id,取id
// phoneNumber代表当前app登录的用户的注册手机号
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
```
### 2.使用service获取本地通讯录列表
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 获取通讯录列表(不传参数默认获取全部)
chatService.listMyContacts().then(contacts=>{
console.log(contacts);
});
// 获取通讯录列表(通过名字检索)
chatService.listMyContacts({contactName:?}).then(contacts=>{
console.log(contacts);
});
// 获取通讯录列表(只获取已经正式成为好友关系的,status参数参考本地通讯录表结构定义)
chatService.listMyContacts({status:5}).then(contacts=>{
console.log(contacts);
});
// 查询我的单个通讯录好友(参数是通讯录contactUid)
chatService.getMyContactById(?).then(contact=>{
console.log(contact);
});
// 查询我的单个通讯录好友(参数是会话id)
chatService.getMyContactByChannelId(?).then(contact=>{
console.log(contact);
});
```
### 3.使用service获取本地会话列表
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 获取会话列表(只查询正常显示的数据,status参数参考本地会话表结构定义)
chatService.listMyChannels({status:1}).then(channels=>{
console.log(channels);
});
// 查询我的单个会话(参数是会话id)
chatService.getMyChannelById(?).then(channel=>{
console.log(channel);
});
```
### 4.使用service获取本地会话的消息记录列表
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 分页查询单个会话对应的消息列表
chatService.listMyMessagesByPage({
pageNumber, // 当前页号
pageSize, // 当前页条数
channelId // 会话id
}).then(page=>{
console.log(page);
})
```
### 5.发送好友申请
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 发送好友申请
// 参数是被申请人的信息
chatService.sendFriendApply({
friendId, // 被申请人id
friendAccountId, // 被申请人账户id
friendPhoneNum, // 被申请人注册手机号
friendName, // 被申请人名称
friendAvatar, //被申请人头像
friendType, // 被申请人类型 0客户 1 门店 2 技师
reason // 申请理由
}).then(contact=>{
//contact是本地通讯录数据,状态为3-本人发起申请
console.log(contact)
})
```
### 6.发送同意好友申请
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 发送同意好友申请的消息
// 参数是申请人的信息(本地通讯录中可以获取)
chatService.sendFriendAgree({
friendId, // 申请人id
friendAccountId, // 申请人账户id
friendPhoneNum, // 申请人注册手机号
friendName, // 申请人名称
friendAvatar, // 申请人头像
friendType, // 申请人类型 0客户 1 门店 2 技师
friendChannelId // 与申请人的唯一会话id
yes, // 是否同意 1-同意 0-拒绝
}).then(({channelLocal,contactLocal})=>{
//contactLocal是本地通讯录数据,状态为5-双方同意
//channelLocal是本地会话数据,状态为2-隐藏
console.log(channelLocal,contactLocal)
})
```
### 7.与好友发送消息(聊天)
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 与好友发送消息
// 参数是双方信息以及消息内容
chatService.sendMessage({
channelId, // 双方之间的唯一会话id
channelType, // 会话类型 1-单聊 2-群聊
from : { // 发送人信息(发送消息时必填)
id, // 发送人id
name, // 发送人名称
avatar // 发送人头像
},
to : { // 接收人信息(发送消息时必填)
id, // 接收人id
name, // 接收人名称
avatar // 接收人头像
},
content, // 消息内容(消息内容需参考`消息体约定`章节中的介绍)
msgType // 消息类型(需参考`消息体约定`章节中的介绍)
}).then((msg)=>{
//msg是本地消息表中的数据
console.log(msg)
})
```
### 8.监听好友申请消息
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 定义回调函数
const callback =({data:contact,err})=> {
// contact是经过service处理后的本地通讯录中的数据
// err是异常,当接收发生错误时,err有值; 正常情况下err为空。
console.log(contact)
}
// 添加监听; 当收到消息时,回调该函数
chatService.onFriendApplyReceived(callback);
// 移除监听,一般在页面生命周期结束后移除
// 注意:callback参数建议必传,不传会移除所有页面添加的回调函数
chatService.offFriendApplyReceived(callback);
```
### 9.监听好友通过我的申请消息
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 定义回调函数
const callback =({data:contact,err})=> {
// contact是经过service处理后的本地通讯录中的数据
// 要注意contact中的status字段,status=5代表对方同意,非5是拒绝
// err是异常,当接收发生错误时,err有值; 正常情况下err为空。
console.log(contact)
}
// 添加监听; 当收到消息时,回调该函数
chatService.onFriendAgreeReceived(callback);
// 移除监听,一般在页面生命周期结束后移除
// 注意:callback参数建议必传,不传会移除所有页面添加的回调函数
chatService.offFriendAgreeReceived(callback);
```
### 10.监听收到聊天消息(监听的内容是收到消息时本地新增的消息本身)
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 定义回调函数
const callback =({data:msg,err})=> {
// msg是经过service处理后的本地消息表中的数据
// err是异常,当接收发生错误时,err有值; 正常情况下err为空。
console.log(msg)
}
// 添加监听; 当收到消息时,回调该函数
chatService.onMsgReceived(callback);
// 移除监听,一般在页面生命周期结束后移除
// 注意:callback参数建议必传,不传会移除所有页面添加的回调函数
chatService.offMsgReceived(callback);
```
### 11.监听收到聊天消息(监听的内容是收到消息时的本地会话变化)
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 定义回调函数
const callback =({data:channel,err})=> {
// channel是经过service处理后的本地会话数据(是更新后的最新状态的会话数据)
// err是异常,当接收发生错误时,err有值; 正常情况下err为空。
console.log(channel)
}
// 添加监听; 当收到消息时,回调该函数
chatService.onMsgReceivedChannelChanges(callback);
// 移除监听,一般在页面生命周期结束后移除
// 注意:callback参数建议必传,不传会移除所有页面添加的回调函数
chatService.offMsgReceivedChannelChanges(callback);
```
### 12.更新通讯录联系人信息(同时更新远端和本地信息)
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 更新通讯录联系人信息
chatService.updateMyContactRemoteAndLocalById(
{
contactUid, // 通讯录联系人id(必填)
contactName, // 通讯录联系人名称(更新项,选填)
contactAvatar, // 通讯录联系人头像(更新项,选填)
contactPhoneNum, // 通讯录联系人账号/手机号(更新项,选填)
contactStatus // 通讯录联系人状态(更新项,选填)
}).then(contact=>{
// contact是更新以后的本地通讯录数据
console.log(contact);
});
// 例如:修改名称
chatService.updateMyContactRemoteAndLocalById({contactName:'阿发',contactUid:'123'}).then((contact)=>{
// contact是修改后的本地通讯录数据
console.log(contact);
});
```
### 13.删除单个通讯录联系人信息(同时更新远端和本地信息)
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 删除单个通讯录数据,同时更新远端和本地信息
// 注意:通讯录删除只是将状态修改了,并没有真正的删除
// 俩个参数 contactUid是本地联系人id ; isClearMsg表示是否要清除本地的聊天记录,默认值是true
chatService.deleteMyContactRemoteAndLocalById(contactUid,isClearMsg).then(contact=>{
// contact是更新状态以后的本地通讯录数据
console.log(contact);
});
```
### 14.更新本地会话信息
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 更新本地会话信息
chatService.updateMyChannelById(
{
id, // 会话id(必填)
title, // 会话标题(更新项,可选)
img, // 会话主展示图(更新项,可选)
seq, // 会话排序值(更新项,可选)
unreadCount,// 会话未读数(更新项,可选)
status, // 会话状态(更新项,可选)
createAt // 会话创建时间(更新项,可选)
}).then(channel=>{
// channel是更新以后的本地会话数据
console.log(channel);
});
```
### 15.删除本地会话(会同时删除会话对应的所有聊天记录)
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 删除本地会话并同时删除会话对应的所有聊天记录
// 注意 会话只是更改了状态,聊天记录是真删除
// 方法接收rest参数 ,可以使用数组也可以直接传入id
// 1.例如使用数组
const channelIds = ['123','456','789'];
chatService.deleteMyChannelsByIds(channelIds).then(()=>{
console.log('删除成功');
});
// 2.例如直接传入id
chatService.deleteMyChannelsByIds('123','456').then(()=>{
console.log('删除成功');
});
chatService.deleteMyChannelsByIds('789').then(()=>{
console.log('删除成功');
});
```
### 16.清除本地会话的未读计数
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 清除本地会话的未读计数
// 方法接收rest参数 ,可以使用数组也可以直接传入id
// 1.例如使用数组
const channelIds = ['123','456','789'];
chatService.clearMyChannelsUnreadCount(channelIds).then(()=>{
console.log('清除成功');
});
// 2.例如直接传入id
chatService.clearMyChannelsUnreadCount('123','456').then(()=>{
console.log('清除成功');
});
chatService.clearMyChannelsUnreadCount('789').then(()=>{
console.log('清除成功');
});
```
### 17.删除本地聊天记录
```javascript
const chatService = ChatService.getInstance({userId,accountId,phoneNumber});
// 删除聊天记录
// 方法接收rest参数 ,可以使用数组也可以直接传入id
// 1. 通过聊天记录的本地自增长id删除
// localIds 是rest参数,不再赘述
chatService.deleteMyMessagesByIds(localIds).then(()=>{
console.log('删除成功');
});
// 2. 通过会话id清空对应会话的聊天记录
// channelIds 是rest参数,不再赘述
chatService.clearMyMessagesByChannelIds(channelIds).then(()=>{
console.log('清除成功');
});
```